Building Microservices with Spring Boot
As software development continues to evolve, the demand for more scalable, flexible, and maintainable systems has increased. One popular approach to achieve this is by adopting microservices architecture. In this blog post, we’ll delve into what microservices are, why they’re beneficial, and how you can implement them using Spring Boot.
What are Microservices?
Microservices are a software development technique that structures an application as a collection of small, independent services. Each service is responsible for a specific business capability or function, and they communicate with each other using lightweight protocols.
Core Principles of Microservices Architecture
- Autonomy: Each service is designed to operate independently, with its own database, APIs, and infrastructure.
- Organized around Business Capabilities: Microservices are built around specific business functions, such as order management or customer support.
- Scale Independently: Each service can scale independently of the others, based on its own traffic patterns.
- Respond Fast to Changes: Microservices can be updated and deployed quickly, without affecting the entire system.
Why Use Microservices?
Microservices offer numerous benefits over traditional monolithic architectures. Some key advantages include:
- Improved Scalability: With microservices, you can scale individual services independently, reducing the need for a massive overhaul of the entire application.
- Increased Flexibility: Microservices allow for more flexibility in technology choices and architecture, making it easier to adapt to changing business requirements.
- Better Resilience: If one service experiences issues, it won’t bring down the entire system. Other services can continue to operate normally.
- Enhanced Maintainability: With microservices, maintenance tasks become much simpler, as you only need to worry about a specific service rather than the entire application.
How to Implement Microservices with Spring Boot
While implementing microservices requires significant effort and planning, Spring Boot provides an excellent framework for building these services. Here’s a high-level overview of how to get started:
- Identify Business Capabilities: Determine which business functions will be handled by individual microservices. For example, you might have separate services for user management, order processing, and inventory management.
- Design Service Interfaces: Define the APIs that each service will expose to communicate with other services. Use RESTful APIs or gRPC for efficient communication.
- Choose Technology Stacks: Select the appropriate technology stacks for each service, considering factors like scalability, performance, and maintainability. Spring Boot is a great choice for Java-based microservices.
- Implement Services: Use Spring Boot to build individual microservices, focusing on specific business capabilities. Leverage Spring Boot’s features like Spring Data for database access, Spring Security for authentication, and Spring Cloud for distributed systems support.
- Integrate Services: Use APIs or messaging queues (e.g., RabbitMQ, Kafka) to integrate services, ensuring seamless communication between them. Implement service discovery with tools like Eureka and load balancing with Ribbon.
Example: Building a Simple Order Management Microservice
Let’s walk through a basic example of creating an order management microservice with Spring Boot:
Create a Spring Boot Project:
Use Spring Initializr to generate a new Spring Boot project with dependencies like Spring Web, Spring Data JPA, and H2 Database.
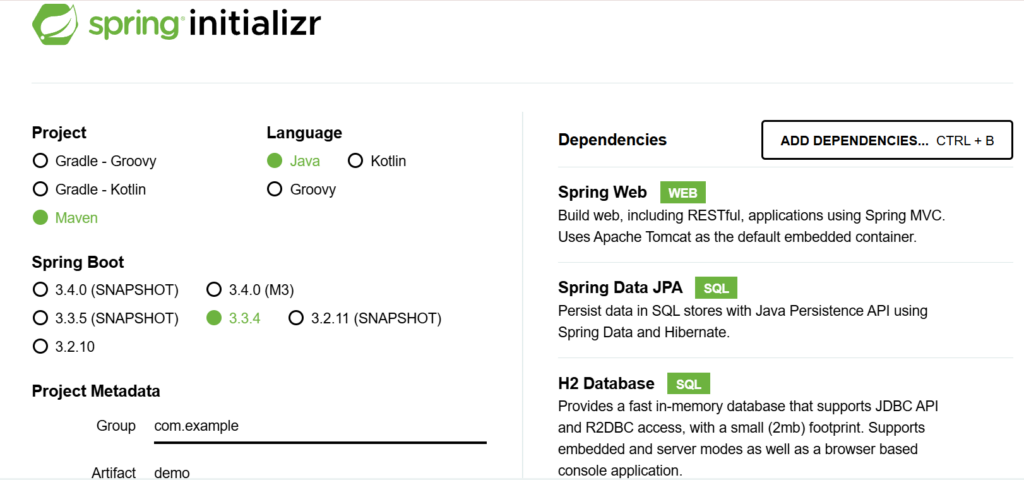
Define the Order Entity:
@Entity
public class Order {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String product;
private int quantity;
private double price;
// Getters and Setters
}
Create the Order Repository:
public interface OrderRepository extends JpaRepository<Order, Long> {
}
Implement the Order Service:
@Service
public class OrderService {
@Autowired
private OrderRepository orderRepository;
public Order createOrder(Order order) {
return orderRepository.save(order);
}
public List<Order> getAllOrders() {
return orderRepository.findAll();
}
}
Expose REST Endpoints:
@RestController
@RequestMapping("/orders")
public class OrderController {
@Autowired
private OrderService orderService;
@PostMapping
public Order createOrder(@RequestBody Order order) {
return orderService.createOrder(order);
}
@GetMapping
public List<Order> getAllOrders() {
return orderService.getAllOrders();
}
}
Conclusion
Building microservices with Spring Boot requires a thoughtful approach, considering factors like scalability, flexibility, and maintainability. By following the principles outlined in this guide, you can create a robust and scalable system that meets your business needs. Remember to identify business capabilities, design service interfaces, choose technology stacks, implement services, and integrate them seamlessly.